C++
OpenGL 4.3
GLSL
WIN32 API
Game Engine
Duration: September 2017 - June 2018 (30 weeks)
​
Created a standalone 3D game/animation engine from scratch using self made libraries (not using STL containers except for offline tools). Project was built in iterations to maintain modularity.
Functional Features:
- Parent-Child-Sibling tree hierarchy and iterator
- Full memory system tracking allocations and deletions in a custom heap using Win32 API
- Robust, custom Math library and functions: Vectors, Matrices, Quaternions, SLERP, and LERP
- File system wrapper over the STL system to add better error reporting and file handling
- Update and Draw loop logic containing various objects for models, textures, shaders, and cameras
- Navigable scene graph accounting for all types of objects in a scene
- Offline converter using the FBX SDK to convert complex models into a format specific for the engine
- Selective keyframe drop compression using a variable delta
- Full 3D model viewer with interactive camera
- Model vertex skinning
- Animation system including keyframes, blending, controllers
- Multiple shader pipeline- Animation, skinning, and blending processed on the GPU using custom compute shaders
Memory system
Created a simple memory system to use heaps, memory allocations, and debug information. Wrapped a system into a library using the WIN32 API.
​
Function Features:
​
- Supports multiple heaps with the ability to create and destroy them
- Allocation from specific heaps
- Per allocation track debug information (file and line number)
- Tracking blocks, which hold tracking information
- Wrapped WIN32 memory system calls for efficiency
- Added debug information to allocations
- Overloaded the new and new[] operators to add more parameters
- Ability to add alignment (game engine is 4 byte aligned)
- Ability to walk and print a list of every allocation to find an arbitrary memory leak
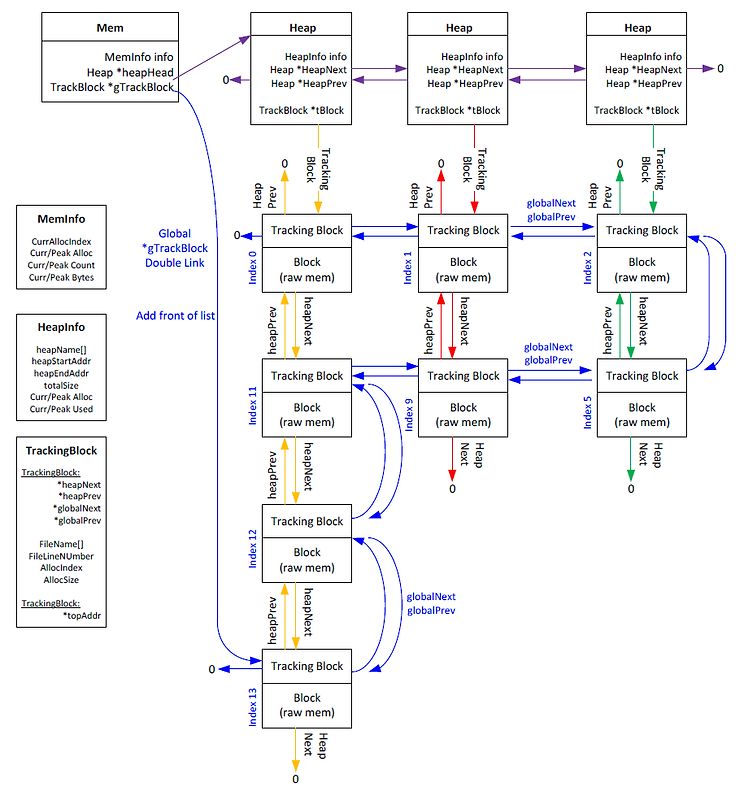
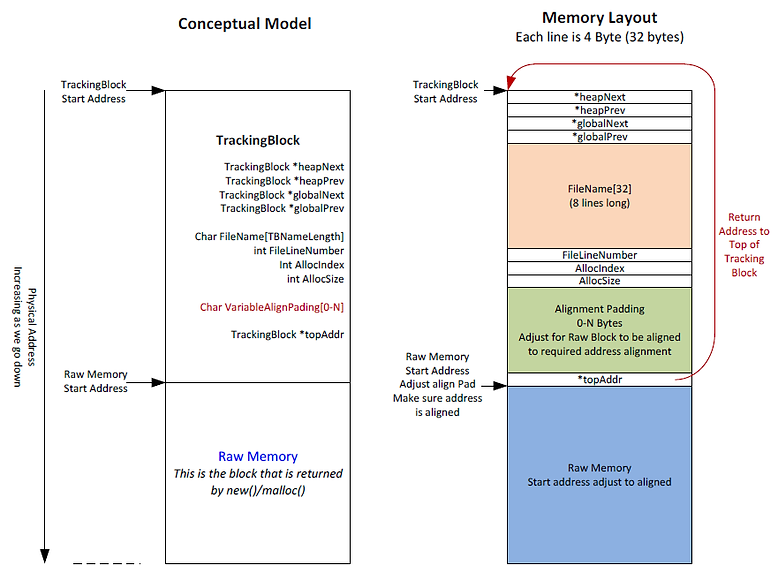
File system
Created a complete file system on top of the WIN32 API, which was integrated within the game engine.
​
Functional Features:
​
- Open/Close file
- Read/Write file
- Flush - flush pending write buffers to the file
- Seek - move to a point in the file
- Handle Based - No direct use of file pointers exposed to the user of the system
- Easy and intuitive interface
- Ability to read different types of data (nothing hard coded)
PCS tree
A parent-child-sibling tree was utilized throughout many of the systems for the game engine. The data structure consists of nodes, where each node can have a single parent, multiple children, and up to two siblings. A single root node exists for any given tree. The PCS tree was used to manage the game objects, animations, skeleton, and cameras. Below is an example of how a PCS tree structure would be organized.

math engine
Created a standalone static math library for computing arithmetic within the game engine. The math library contains various methods for Vector, Matrix, and Quaternion calculations.
archiver
Created a standalone generic archiver, which takes raw binary files and converts them into Chunks using the VODKA converter. Each Chunk contains a ChunkHeader, which holds the type, name, size, and hashNum. We then pack all the Chunks together into one Package using the LIU converter. Similarly, each Package has a PackageHeader, which holds the name, version, number of Chunks, and total size of the Package. Finally we use an EAT function to extract one Chunk from a Package. The archiver is used within custom converters to get model data, such as the bone data, animation data, and hierarchy, from an FBX model. The converters utilizes a console command to execute each program, so that it can be used remotely on any FBX file.
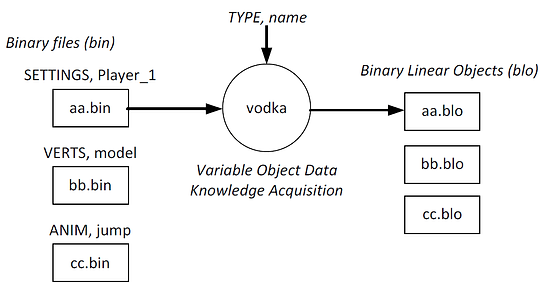
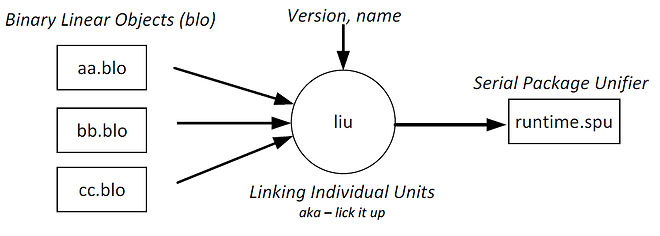
If you're interested in seeing any sample code or executable files, please contact me